Place a ToolstripButton on to the Form :
private void toolStripButton2_Click(object sender, EventArgs e)
{
if (this.ActiveMdiChild == null)
return;
printForm1.Form = this.ActiveMdiChild;
printForm1.PrintAction = System.Drawing.Printing.PrintAction.PrintToPreview;
printForm1.Print();
}
Blog Archive
-
▼
2009
(48)
-
▼
August
(12)
- "PrintForm" Component, available in .NET 3.5 SP1
- How to see Print Preview & Print a WindowsForm
- Deployment of a Windows Forms / Console Application
- Load Text File Rtb
- Load Crystal Report from File
- Export Data from Listview to Excel
- Load Data from Excel File to DataGridView
- DateTime Operations How to get the components of d...
- DateTime Value Manipulation
- How can Reading Excel into Access Database Using A...
- Run Console App From Windows Form Button
- How to Create a File or Folder in C#?
-
▼
August
(12)
My Links
- C# Snippets: 1
- C# Snippets: 2
- XMLFOX - CSHARP SAMPLES
- Q: Export your Crystal Report data to PDF
- Q: To create a Excel report wihtout using Excel object model Ex
- Q: Using C#, How to Save,Update Data to and from XML with out using the sql server database
- Q: upload excel to SQL using C#.net,VS2005
- Q: Export DataTable to Excel using c# with Formatting Styles
- Q: Crystal report in c# .Net
- Q: Import excel into Sql server 2005 Table
- Q: READ, UPDATE & DELETE XML FILE USING X-PATH
- Q: Export Data from Listview to Excel
- Q: About GridView Data To Excel C#
- Q: Simple way to convert excel sheet to sql table
- Q: How to send data from c# file(.cs) to xslt file
- Q: How to Export and import data from sqlserver to excel sheet in vb.net
- Q: Migration of data from access to excel and vice versa
- Q: How to insert the data from excel to Database in .Net?
- Q: How can we create excel sheet in c# using data grid
- Q: DateTime Value Manipulation
- Q: create an array from a txt file
- Q: HowtoOepnandReadanExcelSpreadsheetinaListViewin.NET
- Q: How to fill data to excel
- Q: Reading Image from Excel file using C# .Net
- C# / CSharp Tutorial
- stackoverflow.com/questions
- General .NET Projects
- Sample .NET Projects with source code
- CSharpArticles
- PHPManual
- phpmanual/en/install.windows
- ProtoPage
Sunday, August 23, 2009
"PrintForm" Component, available in .NET 3.5 SP1
How to see Print Preview & Print a WindowsForm
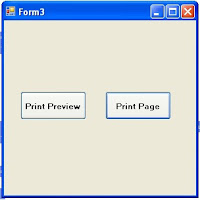
using System.Drawing.Printing; //For "PrintDocument" Class
public partial class Form3 : Form
{
PrintDocument PrintDoc1 = new PrintDocument();
PrintPreviewDialog PPDlg1 = new PrintPreviewDialog();
public Form3()
{
InitializeComponent();
PPDlg1.Document = PrintDoc1;
PrintDoc1.OriginAtMargins =true; //To set or Get the Position of a Graphic Object
PrintDoc1.PrintPage += PDoc_PrintPage;
}
private void btn_PrintPreview_Click(object sender, EventArgs e)
{// When PrintPreview Button Clicks
PPDlg1.ShowDialog();
}
private void PDoc_PrintPage(object sender, PrintPageEventArgs e)
{
Bitmap bmp = new Bitmap(this.Width, this.Height);
//this.DrawToBitmap(bmp, this.ClientRectangle);
this.DrawToBitmap(bmp, new Rectangle(0, 0, bmp.Width, bmp.Height)); //Takes the Snap of the Exact WindowForm size as Bitmap image
e.Graphics.DrawImage(bmp, 0, 0);
}
private void btn_PrintPage_Click(object sender, EventArgs e)
{//When Print Button Clicks, Image will be show & Ready to Print
PrintDoc1.Print();
}
}
Wednesday, August 19, 2009
Deployment of a Windows Forms / Console Application
On the Build menu, choose Rebuild all Projects, to make sure that the client application,
To create a deployment project
1. On the File menu, point to Add, and then click New Project.
2. In the Add New Project dialog box, in the Project Types pane, open the Other Project Types node, click Setup and Deployment Projects, then click Setup Project in the Templates pane. In the Name box, type <project_name>_Setup.
3. Click OK to close the dialog box.
The project is added to Solution Explorer, and the File System Editor opens.
4. Change ProductName property and remove "_Setup" from default ProductName name
· Select the <project_name>_Setup project in Solution Explorer. In the Properties window, select the ProductName property and type <project_name> without _Setup.
Note: · The ProductName property specifies the name that will be displayed for the application in installation folder names and in the Add/Remove Programs dialog box. |
5. Change Manufacturer from ‘Default Company Name’ property to xxx . Then the MSI will install your application installed into the c:\Program Files\xxx\<project_name>\ folder
To add the WinForms / Console application to the installer
1. Right-click the \<project_name>_Setup project in Solution Explorer.
· View -> File System
· In the File System Editor, select the Application Folder node.
2. On the Action menu, point to Add, and then click Project Output.
3. In the Add Project Output Group dialog box:
- choose \<project_name> from the Project drop-down list
- Select the Primary Output group from the list
- In the Configuration box, select (Active).
- Click OK to close the dialog box.
To create Program Files folder & menu
Select the \<project_name>_Setup project in Solution Explorer.
1. Normally Install will, by default, restrict installation to a single user. If needed, in Project Explorer, click Setup Project name, view properties list, and set “InstallAllUsers” to true.
1a. In the File System Editor, right-click User’s Program Menu.
2. Add -> Folder and Rename Folder to <project_name>
3. In the File System Editor, in the Application Folder, select the Primary output from <project_name> node.
4. Right Click -> Create Shortcut to Primary Output.
5. Rename the Shortcut to <project_name>.
6. Select the Shortcut and drag it to your User’s Program Menu folder in the left pane.
To add an icon for Program Files folder & menu
- In the File System Editor, click User’s Program Menu. then in the right panel, right-click the shortcut (not the folder).
In the Properties window, select the Icon property and choose (Browse...) from the drop-down list. The Icon dialog box will be displayed.
In the DropDownListBox, choose "User's Program Menu"
Click the Add File Button, then browse for the Icon file.
The Icon dialog box will be displayed.
In the DropDownListBox, choose "User's Program Menu"
Click the Add File Button, then browse for the Icon file.
Rebuild the _setup project, then you should be ready !
..
Tuesday, August 11, 2009
Load Crystal Report from File
This code will show how to load a crystal report .rpt file that is saved on a local drive instead of embedded. The advantage to this is the program does not need to be re-compiled each time a report is modifed. Also, the .rpt can be upload from the application and stored in a database and then written to file. Do not embed the .rpt file when using this method.
using System;
using CrystalDecisions.CrystalReports.Engine;
using CrystalDecisions.Shared;
namespace Report
{
public partial class Report : Document
{
public void ReportLoad()
{
ReportDocument reportDocument = new ReportDocument();
string filePath = "C:\Projects\Application\Report\CrystalReport.rpt";
reportDocument.Load(filePath);
crystalReportViewer.ReportSource = reportDocument;
}
}
}
..
Export Data from Listview to Excel
private void Export2Excel()
{
try
{
//lvPDF is nothing but the listview control name
string[] st = new string[lvPDF.Columns.Count];
DirectoryInfo di = new DirectoryInfo(@"c:\PDFExtraction\");
if (di.Exists == false)
di.Create();
StreamWriter sw = new StreamWriter(@"c:\PDFExtraction\" + txtBookName.Text.Trim() + ".xls", false);
sw.AutoFlush = true;
for (int col = 0; col < lvPDF.Columns.Count; col++)
{
sw.Write("\t" + lvPDF.Columns[col].Text.ToString());
}
int rowIndex = 1;
int row = 0;
string st1 = "";
for (row = 0; row < lvPDF.Items.Count; row++)
{
if (rowIndex <= lvPDF.Items.Count)
rowIndex++;
st1 = "\n";
for (int col = 0; col < lvPDF.Columns.Count; col++)
{
st1 = st1 + "\t" + "'" + lvPDF.Items[row].SubItems[col].Text.ToString();
}
sw.WriteLine(st1);
}
sw.Close();
FileInfo fil = new FileInfo(@"c:\PDFExtraction\" + txtBookName.Text.Trim() + ".xls");
if (fil.Exists == true)
MessageBox.Show("Process Completed", "Export to Excel", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
catch (Exception ex)
{
}
}
...
Load Data from Excel File to DataGridView
...
To read data from excel file and load that data into DataGridView, this function will take file name as parameter and reads that excel file
Controls Required: DataGridView
using System.Data.OleDb;
using System.IO;
public void setExcelFileAsDataSourceToDataGridView(string FileName)
{
OleDbConnection ocon;
OleDbDataAdapter oda;
DataSet ds;
//Check Whether file is xls file or not
if (Path.GetExtension(FileName) == ".xls")
{
try
{
//Create a OLEDB connection for Excel file
string conStr = "Provider=Microsoft.Jet.OLEDB.4.0;"+"Data Source=" + FileName + ";" + "Extended Properties=Excel 8.0;";
oCon = new OleDbConnection(conStr);
oda = new OleDbDataAdapter("select * from [Sheet1$]",oCon);
ds = new DataSet();
//Fill the Data Set
oda.Fill(ds);
//Set DataSource of DataGridView
dataGridView1.DataSource = ds.Tables[0];
ds.Dispose();
oda.Dispose();
oCon.Dispose();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
else
{
MessageBox.Show("Please select Excel File");
}
}
DateTime Operations How to get the components of date & time (day, month, year, minute, hour, sec, millisec) using “DateTime” Class in C#
* To get the components of the date and time, date time class provides us different properties...
Get Day:
To get day out of date first you will have to get the date after that you can get the day component out of that for this purpose “Date and Time” class provides us a property named Day. This property returns the value of day as an integer.
To demonstrate make a window application. Drag one text box and one button on the form. Press button you will get the result in text box.
Now write the following code on button click event
C#
private void btn_Day_Click(object sender, EventArgs e)
{
DateTime obj = new DateTime();
obj = DateTime.Now;
int a = obj.Day;
string str = a.ToString();
txt_Result1.Text = str;
}
VB
Private Sub btn_Day_Click(ByVal sender As Object, ByVal e As EventArgs)
Dim obj As New DateTime()
obj = DateTime.Now
Dim a As Integer = obj.Day
Dim str As String = a.ToString()
txt_Result1.Text = str
End Sub
This simple code gets the day component out of the date.
Get Month:
To get month out of date first you will have to get the date after that you can get the month component out of that for this purpose “Date and Time” class provides us a property named Month. This property returns the value of month as an integer.
To demonstrate make a window application. Drag one text box and one button on the form. Press button you will get the result in text box.
Now write the following code on button click event
C#
private void btn_Month_Click(object sender, EventArgs e)
{
DateTime obj = new DateTime();
obj = DateTime.Now;
int a = obj.Month;
string str = a.ToString();
txt_Result2.Text = str;
}
VB
Private Sub btn_Month_Click(ByVal sender As Object, ByVal e As EventArgs)
Dim obj As New DateTime()
obj = DateTime.Now
Dim a As Integer = obj.Month
Dim str As String = a.ToString()
txt_Result2.Text = str
End Sub
This simple code gets the month component out of the date.
Get Year:
To get year out of date first you will have to get the date after that you can get the year component out of that for this purpose “Date and Time” class provides us a property named Year. This property returns the value of year as an integer.
To demonstrate make a window application. Drag one text box and one button on the form. Press button you will get the result in text box.
Now write the following code on button click event
C#
private void btn_year_Click(object sender, EventArgs e)
{
DateTime obj = new DateTime();
obj = DateTime.Now;
int a = obj.Year;
string str = a.ToString();
txt_Result3.Text = str;
}
VB
Private Sub btn_year_Click(ByVal sender As Object, ByVal e As EventArgs)
Dim obj As New DateTime()
obj = DateTime.Now
Dim a As Integer = obj.Year
Dim str As String = a.ToString()
txt_Result3.Text = str
End Sub
This simple code gets the year component out of the date
Get Hour:
To get hour out of time first you will have to get the time after that you can get the hour component out of that for this purpose “Date and Time” class provides us a property named Hour. This property returns the value of hours as an integer.
To demonstrate make a window application. Drag one text box and one button on the form. Press button you will get the result in text box.
Now write the following code on button click event
C#
private void btn_hour_Click(object sender, EventArgs e)
{
DateTime obj = new DateTime();
obj = DateTime.Now;
int a = obj.Hour;
string str = a.ToString();
txt_Result4.Text = str;
}
VB
Private Sub btn_hour_Click(ByVal sender As Object, ByVal e As EventArgs)
Dim obj As New DateTime()
obj = DateTime.Now
Dim a As Integer = obj.Hour
Dim str As String = a.ToString()
txt_Result4.Text = str
End Sub
This simple code gets the hour’s component out of the time
Get Minute:
To get minute out of time first you will have to get the time after that you can get the minute component out of that for this purpose “Date and Time” class provides us a property named minute. This property returns the value of minutes as an integer.
To demonstrate make a window application. Drag one text box and one button on the form. Press button you will get the result in text box.
Now write the following code on button click event
C#
private void btn_minute_Click(object sender, EventArgs e)
{
DateTime obj = new DateTime();
obj = DateTime.Now;
int a = obj.Minute;
string str = a.ToString();
txt_Result5.Text = str;
}
VB
Private Sub btn_minute_Click(ByVal sender As Object, ByVal e As EventArgs)
Dim obj As New DateTime()
obj = DateTime.Now
Dim a As Integer = obj.Minute
Dim str As String = a.ToString()
txt_Result5.Text = str
End Sub
This simple code gets the minute’s component out of the time.
Get Second:
To get second out of time first you will have to get the time after that you can get the second’s component out of that for this purpose “Date and Time” class provides us a property named second. This property returns the value of seconds as an integer.
To demonstrate make a window application. Drag one text box and one button on the form. Press button you will get the result in text box.
Now write the following code on button click event
C#
private void btn_second_Click(object sender, EventArgs e)
{
DateTime obj = new DateTime();
obj = DateTime.Now;
int a = obj.Second;
string str = a.ToString();
txt_Result6.Text = str;
}
VB
Private Sub btn_second_Click(ByVal sender As Object, ByVal e As EventArgs)
Dim obj As New DateTime()
obj = DateTime.Now
Dim a As Integer = obj.Second
Dim str As String = a.ToString()
txt_Result6.Text = str
End Sub
This simple code gets the second’s component out of the time.
Get Milliseconds:
To get millisecond out of time first you will have to get the time after that you can get the millisecond component out of that for this purpose “Date and Time” class provides us a property named millisecond. This property returns the value of milliseconds as an integer.
To demonstrate make a window application. Drag one text box and one button on the form. Press button you will get the result in text box.
C#
private void button1_Click(object sender, EventArgs e)
{
DateTime obj = new DateTime();
obj = DateTime.Now;
int a = obj.Millisecond;
string str = a.ToString();
txt_Result7.Text = str;
}
VB
Private Sub button1_Click(ByVal sender As Object, ByVal e As EventArgs)
Dim obj As New DateTime()
obj = DateTime.Now
Dim a As Integer = obj.Millisecond
Dim str As String = a.ToString()
txt_Result7.Text = str
End Sub
This simple code gets the millisecond’s component out of the time.
Now write the following code on FORM LOAD event:
C#
private void Form1_Load(object sender, EventArgs e)
{
this.Text = "Devasp.Net Application";
}
VB
Private Sub Form1_Load(ByVal sender As Object, ByVal e As EventArgs)
Me.Text = "Devasp.Net Application"
End Sub
This simple article tells how we can get the components of date and time (day, month, year, minute, hour, second, millisecond) using “Date Time” Class” in C# and VB.
DateTime Value Manipulation
TimeSpan:
In the following examples, a TimeSpan value is created as required using the new keyword and a TimeSpan constructor.
DateTime theDate = DateTime.Parse("2 Jan 2007 20:15:00");
// Add one hour, one minute and one second
theDate = theDate + new TimeSpan(1, 1, 1); // theDate = 2 Jan 2007 21:16:01
// Subtract twenty-five days
theDate = theDate - new TimeSpan(25, 0, 0, 0);// theDate = 8 Dec 2006 21:16:01
Using ToString with Format Specifiers:
This format specifier permits the use of a wider range of date and time styles.
It does, however, limit the user's control over how they view an application's output.
DateTime theDate = DateTime.Parse("3 Jan 2007 21:25:30");
string result;
result = theDate.ToString("d"); // result = "03/01/2007"
result = theDate.ToString("f"); // result = "03 January 2007 21:25"
result = theDate.ToString("y"); // result = "January 2007"
Available Format Specifiers
The full list of format specifiers for DateTime conversion is as follows:
Specifier Description Example
d Short date format. This is equivalent to using ToShortDateString."03/01/2007"
D Long date format. This is equivalent to using ToLongDateString. "03 January 2007"
f Date and time using long date and short time format. "03 January 2007 21:25"
F Date and time using long date and time format. "03 January 2007 21:25:30"
g Date and time using short date and time format. "03/01/2007 21:25"
G Date and time using short date and long time format. "03/01/2007 21:25:30"
m Day and month only. "03 January"
r Date and time in standard Greenwich Mean Time (GMT) format. "Wed, 03 Jan 2007 21:25:30 GMT"
s Sortable date and time format. The date elements start at the highest magnitude (year) and reduce along the string to the smallest magnitude (seconds). "2007-01-03T21:25:30"
t Short time format. This is equivalent to using ToShortTimeString."21:25"
T Long time format. This is equivalent to using ToLongTimeString. "21:25:30"
u Short format, sortable co-ordinated universal time. "2007-01-03 21:25:30Z"
U Long format date and time. "03 January 2007 17:25:30"
y Month and year only. "January 2007"
Available Picture Formatting Codes:
The above example shows several formats and the results. The full list of available formatting codes is as follows:
Specifier Description Examples
y One-digit year. If the year cannot be specified in one digit then two digits are used automatically. "7"
"95"
yy Two-digit year with leading zeroes if required. "07"
yyyy Full four-digit year. "2007"
g or gg Indicator of Anno Domini (AD). "A.D."
M One-digit month number. If the month cannot be specified in one digit then two digits are used automatically. "1"
"12"
MM Two-digit month number with leading zeroes if required."01"
MMM Three letter month abbreviation. "Jan"
MMMM Month name. "January"
d One-digit day number. If the day cannot be specified in one digit then two digits are used automatically. "3"
"31"
dd Two-digit day number with leading zeroes if required."03"
ddd Three letter day name abbreviation. "Wed"
dddd Day name. "Wednesday"
h One-digit hour using the twelve hour clock. If the hour cannot be specified in one digit then two digits are used automatically. "9" "12"
hh Two-digit hour using the twelve hour clock with leading zeroes if required. "09"
H One-digit hour using the twenty four hour clock. If the hour cannot be specified in one digit then two digits are used automatically. "1" "21"
HH Two-digit hour using the twenty four hour clock with leading zeroes if required. "09"
t Single letter indicator of AM or PM, generally for use with twelve hour clock values. "A"
"P"
tt Two letter indicator of AM or PM, generally for use with twelve hour clock values. "AM"
"PM"
m One-digit minute. If the minute cannot be specified in one digit then two digits are used automatically. "1"
"15"
mm Two-digit minute with leading zeroes if required. "01"
s One-digit second. If the second cannot be specified in one digit then two digits are used automatically. "1"
"59"
ss Two-digit second with leading zeroes if required. "01"
f Fraction of a second. Up to seven f's can be included to determine the number of decimal places to display. "0"
"0000000"
z One-digit time zone offset indicating the difference in hours between local time and UTC time. If the offset cannot be specified in one digit then two digits are used automatically. "+6"
"-1"
zz Two-digit time zone offset indicating the difference in hours between local time and UTC time with leading zeroes if required. "+06"
DateTime theDate = DateTime.Parse("3 Jan 2007 21:25:30");
string result;
result = theDate.ToString("d"); // result = "03/01/2007"
result = theDate.ToString("%d"); // result = "3"
ex: string DMYformat = DateTime.Today.ToString("dd-MM-yyyy"); //11-08-2009
..
How can Reading Excel into Access Database Using ADO.Net & C#
This articles basically describes following things:-
1. Creating Access database from C# code
2. Reading Excel file into database
3. Drop table in Access database using code
4. Create table in Access database using code
5. Copy values from dataset to access table
To start with we will first create a new access database on the system.Here we are creating it in @"C:\AccessDB\NewMDB.mdb".
First of all in your project, select References, and then set references to Microsoft ADO Ext. for DDL and Security and Microsoft ActiveX Data Objects Library. This sample code works with both ADO 2.5 and ADO 2.6, so select the version appropriate to your computer.
As a first step we will create an access database
DataSet ds = new DataSet();
System.Collections.ArrayList tableNames = new System.Collections.ArrayList();
try
{
//Create Access database Start
if (!System.IO.File.Exists(@"C:\AccessDB\NewMDB.mdb"))
{
ADOX.CatalogClass cat = new ADOX.CatalogClass();
cat.Create("Provider=Microsoft.Jet.OLEDB.4.0;" +
"Data Source=C:\\AccessDB\\NewMDB.mdb;" +
"Jet OLEDB:Engine Type=5");
Console.WriteLine("Database Created Successfully");
cat = null;
}
//Create Access database End
}
catch (Exception ex)
{
Console.WriteLine("Database creation failed due to following reason.");
Console.WriteLine(ex.Message);
}
Now we will read the excel file into dataset.For this you just need to make a change in connection string of OledbProvider i.e. to include excel file name instead of database in datasource propoerty and then append Extended Properties=""Excel 8.0;HDR=YES;"".For this particular sample the excel file that I have has three columns viz ID,City and State, you can also do a Select * on excel file.In the select query you can give the name of the excel worksheet to retrieve data from it.
//Read Excel into dataset start
try
{
string connectionString = @"Provider=Microsoft.Jet.OLEDB.4.0;Data Source=Book1.xls;Extended Properties=""Excel 8.0;HDR=YES;""";
DbProviderFactory factory = DbProviderFactories.GetFactory("System.Data.OleDb");
DbDataAdapter adapter = factory.CreateDataAdapter();
DbCommand selectCommand = factory.CreateCommand();
selectCommand.CommandText = "SELECT ID,City,State FROM [Sheet1$]";//Here Sheet1 is worksheet name
DbConnection connection = factory.CreateConnection();
connection.ConnectionString = connectionString;
selectCommand.Connection = connection;
adapter.SelectCommand = selectCommand;
adapter.Fill(ds, "Test");
if (connection.State != ConnectionState.Closed)
{
connection.Close();
}
}
catch (Exception ex)
{
Console.WriteLine("Excel file can't be read.");
Console.WriteLine(ex.Message);
}
finally
{
}
//Read Excel into dataset End
The requirement is that I need to change data everytime so I am dropping the table here using code below.
//Check whether table existe in database if yes Drop it
try
{
if (ds.Tables.Count > 0)
{
ADOX.CatalogClass cat = new ADOX.CatalogClass();
ADODB.Connection con1 = new ADODB.Connection();
try
{
object obj1 = new object();
con1.Open("Provider=Microsoft.Jet.OLEDB.4.0;Data Source=C:\\AccessDB\\NewMDB.mdb;", "", "", 0);
cat.ActiveConnection = con1;
for (int i = 0; i < cat.Tables.Count; i++)
{
tableNames.Add(cat.Tables[i].Name);
}
if (tableNames.Contains("Test"))
{
//Drop table
con1.Execute("Drop table Test", out obj1, 0);
}
}
catch (Exception ex)
{
throw ex;
}
finally
{
if (con1.State != 0)
con1.Close();
cat = null;
con1 = null;
}
}
}
catch (Exception ex)
{
Console.WriteLine("Not able to drop table from database.");
Console.WriteLine(ex.Message);
}
//Check whether table existe in database if yes Drop it
Now that we have dropped the table we can create a new table in database by reading the information from the excel file.As a first baby step lets first create a table with schema only.We will fill the data later on using dataset.
We are iterating the columns from dataset to create a create table query which we will fire in the database to create the table.This is just one off way you get try many other ways also for this.
//Create Access database Table from excel Start
ADODB.Connection con = new ADODB.Connection();
try
{
object obj = new object();
con.Open("Provider=Microsoft.Jet.OLEDB.4.0;Data Source=C:\\AccessDB\\NewMDB.mdb;", "", "", 0);
string sql = "CREATE TABLE Test(";
int cnt = 0;
foreach (DataColumn dc in ds.Tables[0].Columns)
{
sql += dc.ColumnName;
if (dc.DataType == typeof(double))
{
sql += " NUMBER NULL";
}
else
{
sql += " STRING(120) NULL";
}
cnt++;
if (cnt != ds.Tables[0].Columns.Count)
{
sql += ",";
}
}
sql += ")";
con.Execute(sql, out obj, 0);
Console.WriteLine("A new table named Test has been created");
con.Close();
}
catch (Exception ex)
{
Console.WriteLine("Not able to create a new table in database.");
}
finally
{
if (con.State != 0)
{
con.Close();
}
}
//Create Access database Table from excel End
Now as a last part we are going to insert the data into the table that we have just created.For this particular sample I will create Insert queries for each row as there might be some rows in excel that contain invalid data.We need to ignore that.
//Insert into access database start
OleDbConnection oledbCon = new OleDbConnection("Provider=Microsoft.Jet.OLEDB.4.0;Data Source=C:\\AccessDB\\NewMDB.mdb;");
try
{
string insertSql = "INSERT INTO Test (";
string valueClause = "VALUES (";
int cnt = 0;
foreach (DataColumn dc in ds.Tables[0].Columns)
{
insertSql += dc.ColumnName;
valueClause += "@" + dc.ColumnName;
if (cnt != ds.Tables[0].Columns.Count - 1)
{
insertSql += ",";
valueClause += ",";
}
cnt++;
}
insertSql += ")" + valueClause + ")";
try
{
cnt = 0;
foreach (DataRow dr in ds.Tables[0].Rows)
{
OleDbCommand cmd = new OleDbCommand();//"Select * from Test",oledbCon);
cmd.Connection = oledbCon;
cmd.CommandText = insertSql;
foreach (DataColumn dc in ds.Tables[0].Columns)
{
string columnName = dc.ColumnName;
string paramName = "@" + dc.ColumnName;
string paramValue = Convert.ToString(dr[columnName]);
cmd.Parameters.Add(paramName, OleDbType.VarChar);
cmd.Parameters[paramName].Value = paramValue;
}
oledbCon.Open();
cmd.ExecuteNonQuery();
oledbCon.Close();
}
}
catch (Exception ex)
{
if (oledbCon.State == ConnectionState.Open)
oledbCon.Close();
MessageBox.Show(ex.Message);
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
Run Console App From Windows Form Button
When the button is click I want to run this without a console window popping up:
Code Snippet
static void WalkDirectoryTree(System.IO.DirectoryInfo root)
{
System.IO.FileInfo[] files = null;
System.IO.DirectoryInfo[] subDirs = null;
// First, process all the files directly under this folder
try
{
files = root.GetFiles("*.*");
}
// This is thrown if even one of the files requires permissions greater
// than the application provides.
catch (UnauthorizedAccessException e)
{
// This code just writes out the message and continues to recurse.
// You may decide to do something different here. For example, you
// can try to elevate your privileges and access the file again.
log.Add(e.Message);
}
catch (System.IO.DirectoryNotFoundException e)
{
Console.WriteLine(e.Message);
}
if (files != null)
{
foreach (System.IO.FileInfo fi in files)
{
// In this example, we only access the existing FileInfo object. If we
// want to open, delete or modify the file, then
// a try-catch block is required here to handle the case
// where the file has been deleted since the call to TraverseTree().
Console.WriteLine(fi.FullName);
}
// Now find all the subdirectories under this directory.
subDirs = root.GetDirectories();
foreach (System.IO.DirectoryInfo dirInfo in subDirs)
{
// Resursive call for each subdirectory.
WalkDirectoryTree(dirInfo);
}
}
}
How to Create a File or Folder in C#?
public class CreateFileOrFolder
{
static void Main()
{
// Specify a "currently active folder"
string activeDir = @"c:\testdir2";
//Create a new subfolder under the current active folder
string newPath = System.IO.Path.Combine(activeDir, "mySubDir");
// Create the subfolder
System.IO.Directory.CreateDirectory(newPath);
// Create a new file name. This example generates
// a random string.
string newFileName = System.IO.Path.GetRandomFileName();
// Combine the new file name with the path
newPath = System.IO.Path.Combine(newPath, newFileName);
// Create the file and write to it.
// DANGER: System.IO.File.Create will overwrite the file
// if it already exists. This can occur even with
// random file names.
if (!System.IO.File.Exists(newPath))
{
using (System.IO.FileStream fs = System.IO.File.Create(newPath))
{
for (byte i = 0; i < 100; i++)
{
fs.WriteByte(i);
}
}
}
// Read data back from the file to prove
// that the previous code worked.
try
{
byte[] readBuffer = System.IO.File.ReadAllBytes(newPath);
foreach (byte b in readBuffer)
{
Console.WriteLine(b);
}
}
catch (System.IO.IOException e)
{
Console.WriteLine(e.Message);
}
// Keep the console window open in debug mode.
System.Console.WriteLine("Press any key to exit.");
System.Console.ReadKey();
}
}
=>If the folder already exists, CreateDirectory does nothing, and no exception is thrown. However, File.Create does overwrite any existing file. To avoid overwriting an existing file, you can use the OpenWrite()()() method and specify the FileMode.OpenOrCreate option that will cause the file to be appended to rather than overwritten.
The following conditions may cause an exception:
* The folder name is malformed. For example, it contains illegal characters or is only white space ( ArgumentException class). Use the Path class to create valid path names.
* The parent folder of the folder to be created is read-only ( IOException class).
* The folder name is null ( ArgumentNullException class).
* The folder name is too long ( PathTooLongException class).
* The folder name is only a colon, ":" ( PathTooLongException class).
...